#requires -version 2.0
<#
-----------------------------------------------------------------------------
Script: EventLogMorningReport.ps1
Version: 1.0
Author: Jeffery Hicks
http://jdhitsolutions.com/blog
http://twitter.com/JeffHicks
http://www.ScriptingGeek.com
Date: 08/01/2012
Keywords:
Comments:
Prepare a morning system status report of event log information only
"Those who forget to script are doomed to repeat their work."
****************************************************************
* DO NOT USE IN A PRODUCTION ENVIRONMENT UNTIL YOU HAVE TESTED *
* THOROUGHLY IN A LAB ENVIRONMENT. USE AT YOUR OWN RISK. IF *
* YOU DO NOT UNDERSTAND WHAT THIS SCRIPT DOES OR HOW IT WORKS, *
* DO NOT USE IT OUTSIDE OF A SECURE, TEST SETTING. *
****************************************************************
-----------------------------------------------------------------------------
#>
<#
.Synopsis
Create Eventlog Morning Report
.Description
Create an eventlog summary for recent errors and warnings from the System and
Application event logs plus audit failures from the Security event log. The
report by default is sent to the pipeline as a custom object. But you can also
use -TEXT to write a formatted text report, suitable for sending to a file or
printer, or -HTML to create HTML code. You will need to pipe these results to
Out-File if you want to save it.
This script is based on the more comprehensive Morning Report script which you
can find at http://jdhitsolutions.com/blog/2012/02/morning-report-revised
.Parameter Computername
The name of the computer to query. The default is the localhost.
.Parameter ReportTitle
The title for your report. This parameter has an alias of 'Title'.
.Parameter Hours
The number of hours to search for errors, warnings and audit failures.
The default is 24.
.Parameter HTML
Create HTML report. You must pipe to Out-File to save the results.
.Parameter Text
Create a formatted text report. You must pipe to Out-File to save the results.
.Example
PS C:\Scripts\> .\EventlogMorningReport.ps1 | Export-Clixml ("c:\work\{0:yyyy-MM-dd}_{1}.xml" -f (get-date),$env:computername)
Preparing morning report for SERENITY
...System Event Log Error/Warning since 01/09/2012 09:47:26
...Application Event Log Error/Warning since 01/09/2012 09:47:26
Run an eventlog morning report for the local computer and export it to an XML file with a date stamped file name.
.Example
PS C:\Scripts\> .\EventlogMorningReport.ps1 Quark -Text | Out-file c:\work\quark-report.txt
Run an eventlog morning report for a remote computer and save the results to an text file.
.Example
PS C:\Scripts\> .\EventlogMorningReport.ps1 -html -hours 30 | Out-file C:\work\MyReport.htm
Run an eventlog morning report for the local computer and get last 30 hours of event log information. Save as an HTML report.
.Example
PS C:\Scripts\> get-content computers.txt | .\EventLogMorningreport.ps1 -html | out-file c:\work\morningreport.htm
Get the list of computers and create a single HTML report without the event log information.
.Link
Get-EventLog
ConvertTo-HTML
.Inputs
String
.Outputs
Custom object, text or HTML code
#>
[cmdletbinding(DefaultParameterSetName="object")]
Param(
[Parameter(Position=0,ValueFromPipeline=$True,ValueFromPipelineByPropertyName=$True)]
[ValidateNotNullOrEmpty()]
[string]$Computername=$env:computername,
[ValidateNotNullOrEmpty()]
[alias("title")]
[string]$ReportTitle="EventLog Morning Report",
[ValidateScript({$_ -ge 1})]
[int]$Hours=24,
[Parameter(ParameterSetName="HTML")]
[switch]$HTML,
[Parameter(ParameterSetName="TEXT")]
[switch]$Text
)
Begin {
Write-Verbose "Begin $($myinvocation.mycommand)"
#script internal version number used in output
[string]$reportVersion="1.0"
Write-Verbose "Creating report version $reportversion"
if ($html) {
Write-Verbose "Defining html head"
<#
define some HTML style
here's a source for HTML color codes
http://www.immigration-usa.com/html_colors.html
the code must be left justified
#>
$head = @"
$ReportTitle
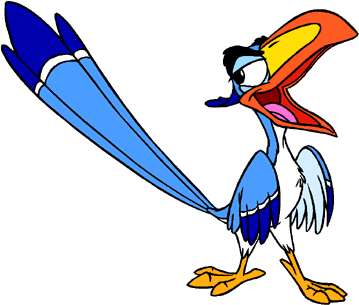
$ReportTitle
$(Get-Date -DisplayHint Date | out-string)
"@
#prepare HTML code
$fragments=@()
} #if $html
} #Begin
Process {
#set a default value for the ping test
$ok=$False
Write-Verbose "Testing if $computername is local"
If ($computername -eq $env:computername) {
#local computer so no ping test is necessary
$OK=$True
Write-Verbose "Local"
}
elseIf (($computername -ne $env:computername) -AND (Test-Connection -ComputerName $computername -quiet -Count 2)) {
#not local computer and it can be pinged so proceed
$OK=$True
Write-Verbose "Pinged remote computer"
}
If ($OK) {
Write-Verbose "OK"
Try {
#use WMI to further test the connection to the computer since Get-Eventlog uses it.
$os=Get-WmiObject Win32_operatingSystem -ComputerName $computername -ErrorAction Stop
#set a variable to indicate WMI can be reached.
$wmi=$True
}
Catch {
Write-Warning "WMI failed to connect to $($computername.ToUpper())"
}
if ($wmi) {
Write-Host "Preparing morning report for $($os.CSname)" -ForegroundColor Cyan
#Event log errors and warnings in the last $Hours hours
$last=(Get-Date).AddHours(-$Hours)
Write-Verbose "Getting event log entries since $last"
#System Log
Write-Host "...System Event Log Error/Warning since $last" -ForegroundColor Cyan
$syslog=Get-EventLog -LogName System -ComputerName $computername -EntryType Error,Warning -After $last -ErrorAction SilentlyContinue
$syslogdata=$syslog | Select TimeGenerated,EventID,Source,Message
#Application Log
Write-Host "...Application Event Log Error/Warning since $last" -ForegroundColor Cyan
$applog=Get-EventLog -LogName Application -ComputerName $computername -EntryType Error,Warning -After $last -ErrorAction SilentlyContinue
$applogdata=$applog | Select TimeGenerated,EventID,Source,Message
#Security log
Write-Host "...Security Event Log FailureAudit since $last" -ForegroundColor Cyan
$seclog=Get-EventLog -LogName Security -ComputerName $computername -EntryType FailureAudit -After $last -ErrorAction SilentlyContinue
$seclogdata=$seclog | Select TimeGenerated,EventID,Source,Message
} #if wmi is ok
#write results depending on parameter set
$footer="Report v{3} run {0} by {1}\{2}" -f (Get-Date),$env:USERDOMAIN,$env:USERNAME,$reportVersion
if ($HTML) {
Write-Verbose "HTML"
#add each computer to a navigation menu in the header
$head+=("{0} " -f $computername.ToUpper())
$fragments+=("" -f $computername.ToUpper())
#insert navigation bookmarks
$nav=@"
{0} System Log
{0} Application Log
{0} Security Log
"@ -f $Computername.ToUpper()
#add a link to the document top
$nav+="`nTop"
$fragments+=$nav
$fragments+="
"
$fragments+=$syslogData | ConvertTo-HTML -Fragment -PreContent ("" -f $computername.toUpper())
$fragments+=$nav
$fragments+=$applogData | ConvertTo-HTML -Fragment -PreContent ("" -f $computername.toUpper())
$fragments+=$nav
$fragments+=$seclogData | ConvertTo-HTML -Fragment -PreContent ("" -f $computername.toUpper())
$fragments+=$nav
} #if html
elseif ($TEXT) {
Write-Verbose "Text"
#prepare formatted text
$ReportTitle
"-"*($ReportTitle.Length)
"Eventlog Summary"
Write "System Event Log Summary"
$syslogdata | Format-List | Out-String
Write "Application Event Log Summary"
$applogdata | Format-List | Out-String
Write "Security Event Log Summary"
$seclogdata | Format-List | Out-String
Write $Footer
} #elseif text
else {
Write-Verbose "Object"
#Write data to the pipeline as part of a custom object
New-Object -TypeName PSObject -Property @{
SystemLog=$syslogdata
ApplicationLog=$applogdata
SecurityLog=$seclogdata
ReportVersion=$reportVersion
RunDate=Get-Date
RunBy="$env:USERDOMAIN\$env:USERNAME"
}
} #else
} #if OK
else {
#can't ping computer so fail
Write-Warning "Failed to ping $computername"
}
} #process
End {
Write-Verbose "End $($myinvocation.mycommand)"
#if HTML finish the report here so that if piping in
#computer names we get one report for all computers
If ($HTML) {
#copying fragments to clipboard for troubleshooting
#Write $fragments | clip
$head+="
"
ConvertTo-Html -Head $head -Title $ReportTitle -PreContent ($fragments | out-String) -PostContent "
$footer"
}
Write-Host "Finished!" -ForegroundColor Green
}
#end of script