Earlier this week I did a live session for the PSPowerHour. I talked about ways to dress up your PowerShell work and console sessions. One of things I talked about was using ANSI escape sequences to add some color. What I realized was that I was constantly referring to my reference source at https://en.wikipedia.org/wiki/ANSI_escape_code. That's good up to a point, but I still need to see what a value looks like in my console to know if I want to use it. I don't know why I didn't do this sooner but I wrote a quick and dirty script to display ANSI sequences from a PowerShell prompt. There are others in the wild if you don't like this one.
ManageEngine ADManager Plus - Download Free Trial
Exclusive offer on ADManager Plus for US and UK regions. Claim now!
#requires -version 5.1
#requires -module PSScriptTools
#Show-ANSI.ps1
#comment out the Add-Border lines if you aren't using the PSScriptTools module.
[cmdletbinding()]
Param(
[switch]$Basic,
[switch]$Foreground,
[switch]$Background,
[int[]]$RGB
)
# a private function to display results in columns on the screen
Function display {
Param([object]$all,[int]$Max)
$c = 1
for ($i=0;$i -le $all.count;$i++) {
if ($c -gt $max) {
Write-Host "`r"
$c=1
}
Write-Host "$($all[$i]) `t" -NoNewline
$c++
}
}
if ($IsCoreCLR) {
$esc = "`e"
$escText = '`e'
$max = 3
}
else {
$esc = $([char]0x1b)
$escText = '$([char]0x1b)'
$max = 2
}
#basic
if ($basic) {
Add-Border "Basic Sequences" -ansitext "$esc[1m"
$basichash = @{
1 = "Bold"
2 = "Faint"
3 = "Italic"
4 = "Underline"
5 = "SlowBlink"
6 = "RapidBlink"
7 = "Reverse"
9 = "CrossedOut"
}
$basichash.GetEnumerator() | ForEach-Object {
$sequence = "$esctext[$($_.name)m$($_.value)$esctext[0m"
#"$esc[$($_.name)m$($_.value)$esc[0m"
"$esc[$($_.name)m$sequence$esc[0m"
}
}
If ($Foreground) {
Add-Border "Foreground" -ansitext "$esc[93m"
$n = 30..37
$n+= 90..97
$all = $n | ForEach-Object {
$sequence = "$esctext[$($_)mHello$esctext[0m"
"$esc[$($_)m$sequence$esc[0m"
}
display -all $all -Max $max
Write-Host "`n"
Add-Border "8-Bit Foreground" -ansitext "$esc[38;5;10m"
$all = 1..255 | ForEach-Object {
$sequence = "$esctext[38;5;$($_)mHello$esctext[0m"
"$esc[38;5;$($_)m$sequence$esc[0m"
}
display -all $all -Max $max
}
If ($Background) {
Add-Border "Background" -ansitext "$esc[46m"
$n = 40..47
$n+= 100..107
$all = $n | ForEach-Object {
$sequence = "$esctext[$($_)mHello$esctext[0m"
"$esc[1;$($_)m$sequence$esc[0m"
}
display -all $all -Max $max
Write-Host "`n"
Add-Border "8-Bit Background" -ansitext "$esc[7;38;5;10m"
$all = 1..255 | ForEach-Object {
$sequence = "$esctext[48;5;$($_)mHello$esctext[0m"
"$esc[1;48;5;$($_)m$sequence$esc[0m"
}
display -all $all -Max $max
}
if ($RGB) {
if ($rgb.count -ne 3) {
Write-Warning "Wrong number of arguments. Specify RED,GREEN and BLUE values"
return
}
$test = $rgb | Where-object {$_ -gt 255}
if ($test.count -gt 0) {
Write-Warning "Detected a value above 255"
return $rgb
}
$r = $rgb[0]
$g = $rgb[1]
$b = $rgb[2]
$sequence = "$esctext[38;2;$r;$g;$($b)m256 Color (R:$r)(G:$g)(B:$b)$esctext[0m"
"$esc[38;2;$r;$g;$($b)m$sequence$esc[0m"
}
As I said, this is a "quick and dirty" solution. The intention was that you could run it in a Windows PowerShell or PowerShell 7 session and see exactly what a sequence looks like on your system. The output will include the exact sequence you would use depending on your PowerShell version.
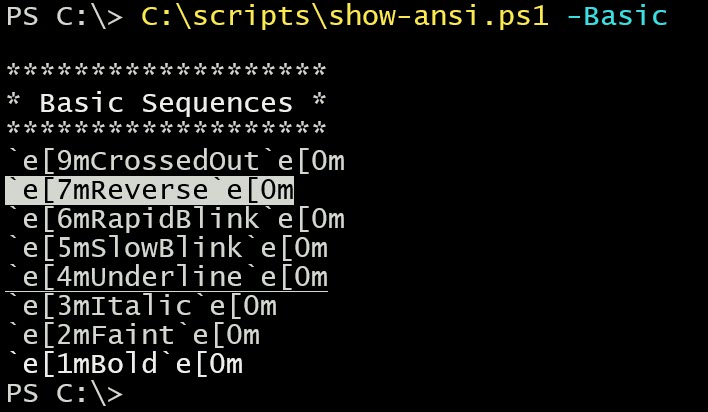
You can test out basic settings. Not every setting will work on every system. For me, it looks like I could use Reverse, Underline and Bold.
You can see Foreground options.
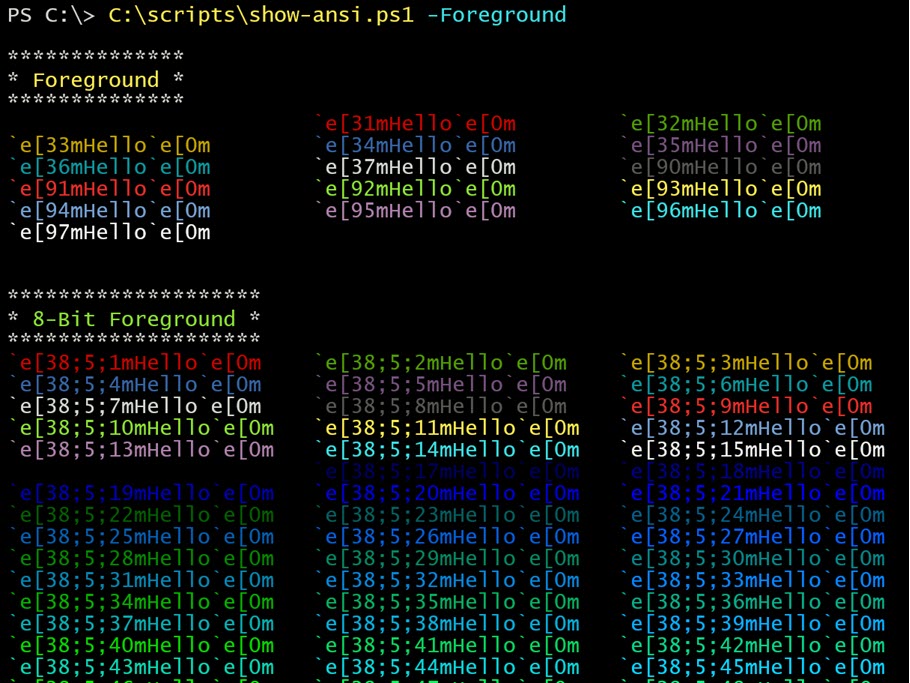
Background options:
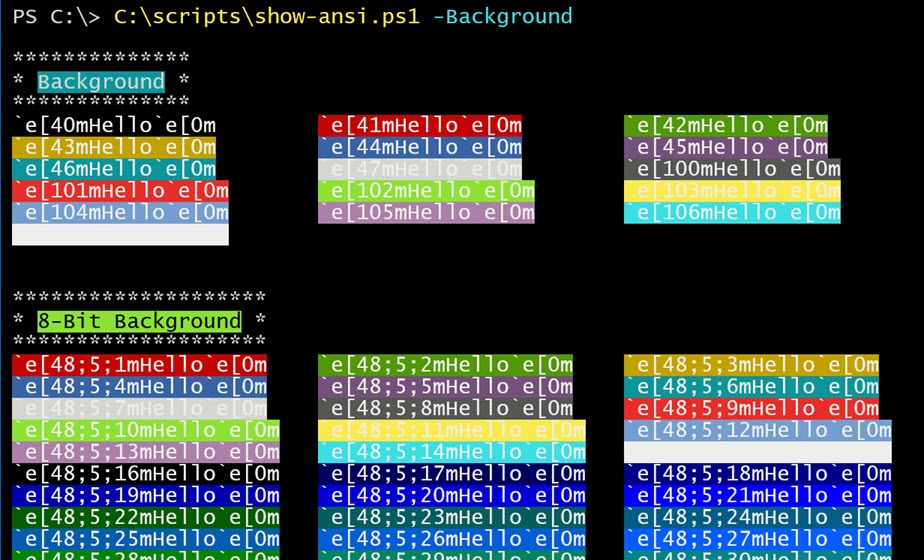
I thought about displaying every RGB combination but realized that would quickly get out of hand. Instead you can specify an array of values in the order of R,G,B.
As you can see, or actually can't, some sequences work better than others depending on your session. Which is one of the reasons I wrote this.
I hope you find it useful.