Time for a new Friday Fun article. These articles use "fun" ways to teach you how to use PowerShell. The end goal may be silly, but hopefully the techniques and concepts are useful. Today I have an updated prompt function. You can customize your PowerShell prompt by creating a function called prompt and loading it into your PowerShell session.
ManageEngine ADManager Plus - Download Free Trial
Exclusive offer on ADManager Plus for US and UK regions. Claim now!
The prompt function only lasts for as long as your PowerShell session is running. Run:
get-content function:prompt
to see your current prompt.
Because Christmas is coming, and given how 2020 has been, we might want something to brighten up our day. I have a PowerShell prompt function that gives you a Christmas countdown, wrapped in emojis and color.
<#
display a colorful Christmas countdown prompt
🎄🌠 Christmas in 17.09:44:21 ⛄✨ PS C:\scripts>
this prompt requires a TrueType font
you would put this in your profile script so that it
only runs in December 1-24
#>
if ((Get-Date).Month -eq 12 -AND (Get-Date).Day -lt 25) {
#dot source the emoji script
. C:\scripts\PSEmoji.ps1
#load the Christmas prompt
Function Prompt {
#get current year
$year = (Get-Date).year
#get a timespan between Christmas for this year and now
$time = [datetime]"25 December $year" - (Get-Date)
#turn the timespan into a string and strip off the milliseconds
$timestring = $time.ToString().Substring(0, 11)
#get random string of decorative characters
#they can be pasted emojis or created from values
$Snow = "❄️"
# $snow = ConvertTo-Emoji 0x2744
# $sparkles = "✨"
$sparkles = ConvertTo-Emoji 0x2728
#$snowman = "⛄"
$snowman = ConvertTo-Emoji 0x26C4
$santa = ConvertTo-Emoji 0x1F385
$mrsClaus = ConvertTo-Emoji 0x1F936
$tree = ConvertTo-Emoji 0x1F384
$present = ConvertTo-Emoji 0x1F381
$notes = ConvertTo-Emoji 0x1F3B5
$bow = ConvertTo-Emoji 0x1F380
$star = ConvertTo-Emoji 127775
$shootingStar = ConvertTo-Emoji 127776
$myChars = $santa, $mrsClaus, $tree, $present, $notes, $bow, $star, $shootingStar, $snow, $snowman,$sparkles
#get a few random elements for the prompt
$front = -join ($myChars | Get-Random -Count 2)
$back = -join ($myChars | Get-Random -Count 2)
#the text to display
$text = "Christmas is coming in $timestring"
#get each character in the text and randomly assign each a color using an ANSI sequence
$colorText = $text.tocharArray() | ForEach-Object {
$i = Get-Random -Minimum 1 -Maximum 50
switch ($i) {
{ $i -le 50 -AND $i -ge 45 } { $seq = "$([char]0x1b)[1;5;38;5;199m" }
{ $i -le 45 -AND $i -ge 40 } { $seq = "$([char]0x1b)[1;5;38;11;199m" }
{ $i -le 40 -AND $i -ge 30 } { $seq = "$([char]0x1b)[1;38;5;50m" }
{ $i -le 20 -and $i -gt 15 } { $seq = "$([char]0x1b)[1;5;38;5;1m" }
{ $i -le 16 -and $i -gt 10 } { $seq = "$([char]0x1b)[1;38;5;47m" }
{ $i -le 10 -and $i -gt 5 } { $seq = "$([char]0x1b)[1;5;38;5;2m" }
default { $seq = "$([char]0x1b)[1;37m" }
}
"$seq$_$([char]0x1b)[0m"
} #foreach
#write the prompt text to the host on its own line
Write-Host "$front $($colortext -join '') $back" #-NoNewline #-foregroundcolor $color
#the function needs to write something to the pipeline
"PS $($executionContext.SessionState.Path.CurrentLocation)$('>' * ($nestedPromptLevel + 1)) "
} #end function
} #If December
You can dot source the script file and if the date is between 12 December and Christmas, it will load a new prompt. The prompt will calculate a timespan indicating how long until Christmas.
$year = (Get-Date).year
#get a timespan between Christmas for this year and now
$time = [datetime]"25 December $year" - (Get-Date)
#turn the timespan into a string and strip off the milliseconds
$timestring = $time.ToString().Substring(0, 11)
The rest of the function is embellishment around this text:
#the text to display
$text = "Christmas is coming in $timestring"
I'm going to display emojis randomly chosen from an array of characters. You can either paste an emoji into your code or use my ConvertTo-Emoji function which converts the hex value. The pasted emojis I'm using may not display properly here so I've included equivalents. Here's the script that is dot sourced in the prompt script.
#These commands work best in a PowerShell session running in Windows Terminal.
# http://www.unicode.org/emoji/charts/full-emoji-list.html
Function ConvertTo-Emoji {
[cmdletbinding()]
[alias("cte")]
Param(
[parameter(Position = 0, Mandatory, ValueFromPipeline, HelpMessage = "Specify a value like 0x1F499 or 128153")]
[int]$Value
)
Process {
if ($env:wt_Session -OR ($host.name -match "studio")) {
[char]::convertfromutf32($Value)
}
else {
Write-Warning 'This command is only supported when running in Windows Terminal at this time.'
}
}
}
Function Show-Emoji {
[cmdletbinding()]
Param(
[parameter(Position = 0, Mandatory,HelpMessage = "Enter the starting Unicode value")]
[int32]$Start,
[Parameter(HelpMessage = "How many items do you want to display?")]
[int]$Count = 20
)
Write-verbose "Starting at $Start and getting $count items"
$counter = 1
Do {
for ($i=1;$i -le 5;$i++) {
write-verbose "Counter = $counter i = $i"
$item = "{0} {1} " -f (ConvertTo-Emoji ($start)),$start
if ($counter -le $count) {
write-host $item -NoNewline
$counter++
$start++
}
}
write-host "`r"
} until ($counter -ge $count)
}
The last part of the prompt is to take the string and display each character with an ANSI escape sequence. I've written the sequence so it will work in both Windows PowerShell and PowerShell 7.
#get each character in the text and randomly assign each a color using an ANSI sequence
$colorText = $text.tocharArray() | ForEach-Object {
$i = Get-Random -Minimum 1 -Maximum 50
switch ($i) {
{ $i -le 50 -AND $i -ge 45 } { $seq = "$([char]0x1b)[1;5;38;5;199m" }
{ $i -le 45 -AND $i -ge 40 } { $seq = "$([char]0x1b)[1;5;38;11;199m" }
{ $i -le 40 -AND $i -ge 30 } { $seq = "$([char]0x1b)[1;38;5;50m" }
{ $i -le 20 -and $i -gt 15 } { $seq = "$([char]0x1b)[1;5;38;5;1m" }
{ $i -le 16 -and $i -gt 10 } { $seq = "$([char]0x1b)[1;38;5;47m" }
{ $i -le 10 -and $i -gt 5 } { $seq = "$([char]0x1b)[1;5;38;5;2m" }
default { $seq = "$([char]0x1b)[1;37m" }
}
"$seq$_$([char]0x1b)[0m"
} #foreach
Some of the sequences include blinking characters. The entire line of text is written to the host.
Write-Host "$front $($colortext -join '') $back"
The prompt concludes using the default location code.
"PS $($executionContext.SessionState.Path.CurrentLocation)$('>' * ($nestedPromptLevel + 1)) "
Originally, I was using Write-Host with -NoNewLine so that the text preceded the location. But I like the look of it on its own line. Want to see?
Save the files to the same directory and then dot source the prompt script.
. c:\scripts\christmasprompt.ps1
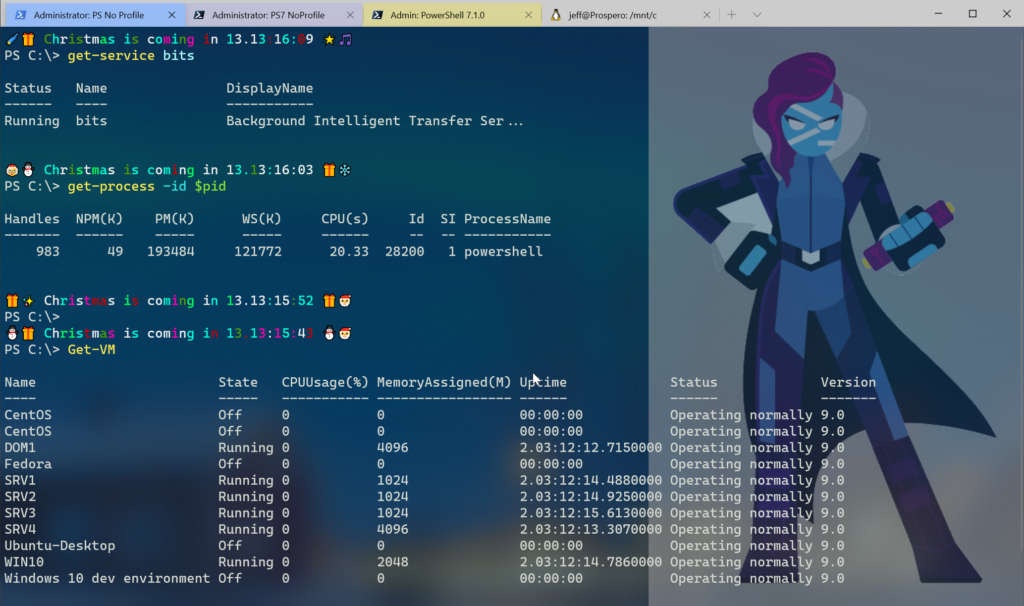
Because the ANSI colors are random, you get something different every time hit Enter. The blinking characters make your prompt sparkle.
Yes, this silly and has no practical purpose, unless you are a terrible procrastinator and need the reminder! But look at the PowerShell techniques: dot sourcing, switch, functions, sub-expressions,timespans, If statements. These are all things you will use in your real PowerShell work. If you are still learning PowerShell, sometimes it helps to see these things demonstrated in different ways.
I hope you'll at least give the code a try. The prompt only lasts for as long as PowerShell is running so there's no risk. Enjoy!