When I'm working on a Pluralsight course, I tend to setup a virtual machine for recording. Although, lately I've been trying with Windows 10 Sandbox. This is handy when all I need is a Windows 10 desktop. When I setup the system, I have particular settings I need to configure. Naturally I use a PowerShell script to automate the process. One item that I wanted to address was Windows 10 taskbar. When I'm recording a course, I like to have it auto-hide. Sure, I could manually set it. But that's no fun.
ManageEngine ADManager Plus - Download Free Trial
Exclusive offer on ADManager Plus for US and UK regions. Claim now!
After a little online research I came across this page. In addition to the manual steps, the author also provided a snippet of PowerShell code! I assumed there would be registry setting I could configure and was hoping to find that. But this was even better. The author's code was written to be used from a CMD prompt to invoke a few PowerShell commands. But since I'm already using PowerShell for my desktop configuration, I took his code and created re-usable PowerShell functions.
The hack is to modify the registry key found at 'HKCU:SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\StuckRects3'. The Settings key is binary. But in PowerShell, it will be shown as an array.
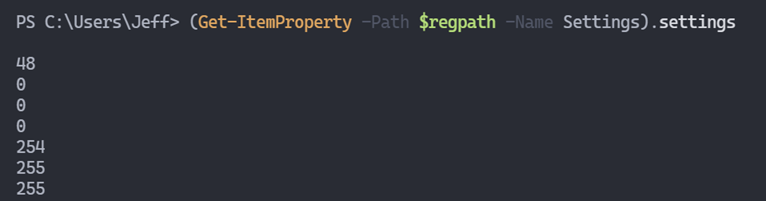
To toggle the auto-hide setting for the Taskbar, all you need to so is set item [8]. A value of 3 will enable auto-hide and 2 will turn it off. There is one caveat, and that is you need to restart the Explorer process for the change to take effect. No problem. I can use Stop-Process. Want to see what I came up with?
#requires -version 5.1
# https://www.howtogeek.com/677619/how-to-hide-the-taskbar-on-windows-10/
Function Enable-AutoHideTaskBar {
#This will configure the Windows taskbar to auto-hide
[cmdletbinding(SupportsShouldProcess)]
[Alias("Hide-TaskBar")]
[OutputType("None")]
Param()
Begin {
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Starting $($myinvocation.mycommand)"
$RegPath = 'HKCU:SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\StuckRects3'
} #begin
Process {
if (Test-Path $regpath) {
Write-Verbose "[$((Get-Date).TimeofDay) PROCESS] Auto Hiding Windows 10 TaskBar"
$RegValues = (Get-ItemProperty -Path $RegPath).Settings
$RegValues[8] = 3
Set-ItemProperty -Path $RegPath -Name Settings -Value $RegValues
if ($PSCmdlet.ShouldProcess("Explorer", "Restart")) {
#Kill the Explorer process to force the change
Stop-Process -Name explorer -Force
}
}
else {
Write-Warning "Can't find registry location $regpath."
}
} #process
End {
Write-Verbose "[$((Get-Date).TimeofDay) END ] Ending $($myinvocation.mycommand)"
} #end
}
Function Disable-AutoHideTaskBar {
#This will disable the Windows taskbar auto-hide setting
[cmdletbinding(SupportsShouldProcess)]
[Alias("Show-TaskBar")]
[OutputType("None")]
Param()
Begin {
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Starting $($myinvocation.mycommand)"
$RegPath = 'HKCU:SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\StuckRects3'
} #begin
Process {
if (Test-Path $regpath) {
Write-Verbose "[$((Get-Date).TimeofDay) PROCESS] Auto Hiding Windows 10 TaskBar"
$RegValues = (Get-ItemProperty -Path $RegPath).Settings
$RegValues[8] = 2
Set-ItemProperty -Path $RegPath -Name Settings -Value $RegValues
if ($PSCmdlet.ShouldProcess("Explorer", "Restart")) {
#Kill the Explorer process to force the change
Stop-Process -Name explorer -Force
}
}
else {
Write-Warning "Can't find registry location $regpath."
}
} #process
End {
Write-Verbose "[$((Get-Date).TimeofDay) END ] Ending $($myinvocation.mycommand)"
} #end
}
Because I'm making changes to the registry and killing a process, the functions have support for -WhatIf. Set-ItemProperty will inherit the WhatIf preference. Technically, so will Stop-Process, but I wanted to demonstrate how you can add your own WhatIf handling for commands that don't recognize -WhatIf.
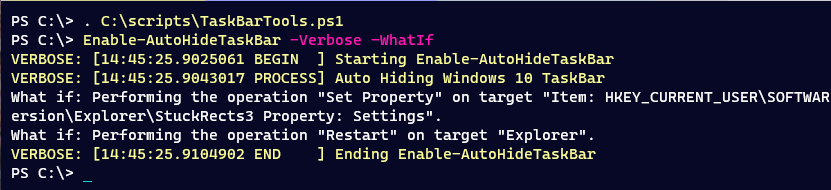
The command is written to work locally and only affects the current user. The functions also have aliases that are clearer, and task specific.
You may not need the commands, but I hope there is a technique or concept you find useful.
Now if you'll excuse me, I have got to get this new course recorded.
Hi Jeff,
Thanks for your sharing.
Do you know any good way to validate a AD user name and password combination is still valid? It is so frustrating to set windows service or IIs pool identity with a wrong paaword withou noticing in powershell.
Kind regards,
yang,
I use this code to test AD credentials:
$Loop = 1
do {
$ADcred = Get-Credential (“$env:USERDOMAIN\$env:USERNAME$”) -Message “Active Directory Credentials”
if (!$ADcred) {
exit
}
$User = $ADcred.Username
$Password = $ADcred.GetNetworkCredential().Password
$CurrentDomain = “LDAP://” + ([ADSI]””).DistinguishedName
$Domain = New-Object System.DirectoryServices.DirectoryEntry($CurrentDomain, $User, $Password)
if ($null -eq $Domain.Name) {
Write-Warning “Authentication failed – Please check user and password!”
$Loop = 1
}
else {
$Loop = 0
}
}
While ($Loop -eq 1)
yang,
I couldn’t manage to make the script to show correctly here…
But maybe you’ll get the idea.
Thank you for sharing your knowledge.
Do you any script that pin or unpin shortcut to taskbar ?. I have tried customized the layout.xml and import-layout etc.. didn’t give me any luck. I know we can do with GPO, but I would like to do with powershell script.
Please share the script if you have.
I think I have some rough code that does this. But it might only work for the current user.
This is not as easy to automate as we might like. The best you can do is create a reference taskbar. Save the .lnk files under C:\Users\\AppData\Roaming\Microsoft\Internet Explorer\Quick Launch\User Pinned\TaskBar so that you can copy them to the same location on a new system. These are user-specific. Then use regedit or reg.exe to export [HKEY_CURRENT_USER\Software\Microsoft\Windows\CurrentVersion\Explorer\Taskband] from the reference machine and then use reg.exe to merge it on the new computer. I don’t think there is a way to handle this on a more granular level.
Jeff
I have always struggled trying to understand what “myinvocation” is and what it does. I have looked it up but it just doesn’t register. Please explain in laymen’s terms or give example that is easy to understand.
Love your work.
Thanks
Phillip
This is a great question. I will work on something for next week.