Yesterday I shared a few PowerShell functions for configuring the Windows 10 taskbar to auto-hide. This works great in my virtual desktop when recording my Pluralsight courses. But even when hidden I would still get an annoying white sliver from the search box. So I got rid of that as well. Here are some PowerShell functions that will hide and unhide the Search box in a Windows 10 desktop. Yes, there are manual steps to hide this feature, but I'm automating here! As always, even if you don't think you need to do this, there might be useful PowerShell nuggets in the code.
ManageEngine ADManager Plus - Download Free Trial
Exclusive offer on ADManager Plus for US and UK regions. Claim now!
The setting that controls the search box is in the registry for the current user. The registry setting is HKCU:\SOFTWARE\Microsoft\Windows\CurrentVersion\Search. Underneath that a key called SearchBoxTaskbarMode controls the display. More than likely this key doesn't exist on your desktop. If the DWord value is 0, the search box will be hidden. A value of 2 will display it. You can use PowerShell to set this value.
Set-ItemProperty -Path HKCU:\SOFTWARE\Microsoft\Windows\CurrentVersion\Search -Name SearchBoxTaskbarMode -Value 0 -Type DWord -Force
The -Force parameter will create the entry if it doesn't exist. As with auto-hiding the taskbar, you will need to restart the Explorer process. Since I'm doing this often it seems, I decided to put this into a separate function. One reason is that when using the Windows Sandbox, sometimes Explorer doesn't automatically restart as it should. My function kills Explorer, tests if it is running and if it isn't starts it.
Function Restart-Explorer {
<#
.Synopsis
Restart the Windows Explorer process.
#>
[cmdletbinding(SupportsShouldProcess)]
[Outputtype("None")]
Param()
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Starting $($myinvocation.mycommand)"
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Stopping Explorer.exe process"
Get-Process -Name Explorer | Stop-Process -Force
#give the process time to start
Start-Sleep -Seconds 2
Try {
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Verifying Explorer restarted"
$p = Get-Process -Name Explorer -ErrorAction stop
}
Catch {
Write-Warning "Manually restarting Explorer"
Try {
Start-Process explorer.exe
}
Catch {
#this should never be called
Throw $_
}
}
Write-Verbose "[$((Get-Date).TimeofDay) END ] Ending $($myinvocation.mycommand)"
}
I then built relatively simple functions to disable and enable the search box.
Function Disable-TaskBarSearch {
<#
.Synopsis
Disable the Windows taskbar search box
#>
[cmdletbinding(SupportsShouldProcess)]
[Alias("Hide-SearchBar")]
[OutputType("Boolean")]
Param()
Begin {
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Starting $($myinvocation.mycommand)"
} #begin
Process {
Write-Verbose "[$((Get-Date).TimeofDay) PROCESS] Hiding Task Bar Search"
Try {
$splat = @{
Path = 'HKCU:\SOFTWARE\Microsoft\Windows\CurrentVersion\Search'
Name = 'SearchBoxTaskbarMode'
Value = 0
Type = 'DWord'
Force = $True
ErrorAction = 'Stop'
}
Set-ItemProperty @splat
if ($WhatIfPreference) {
#return false if using -Whatif
$False
}
else {
$True
}
}
Catch {
$False
Throw $_
}
Restart-Explorer
} #process
End {
Write-Verbose "[$((Get-Date).TimeofDay) END ] Ending $($myinvocation.mycommand)"
} #end
}
Function Enable-TaskBarSearch {
<#
.Synopsis
Enable the Windows taskbar search box
#>
[cmdletbinding(SupportsShouldProcess)]
[Alias("Show-SearchBar")]
[OutputType("Boolean")]
Param()
Begin {
Write-Verbose "[$((Get-Date).TimeofDay) BEGIN ] Starting $($myinvocation.mycommand)"
} #begin
Process {
Write-Verbose "[$((Get-Date).TimeofDay) PROCESS] Showing Task Bar Search"
Try {
$splat = @{
Path = 'HKCU:\SOFTWARE\Microsoft\Windows\CurrentVersion\Search'
Name = 'SearchBoxTaskbarMode'
Value = 2
Type = 'DWord'
Force = $True
ErrorAction = 'Stop'
}
Set-ItemProperty @splat
if ($WhatIfPreference) {
#return false if using -Whatif
$False
}
else {
$True
}
}
Catch {
$False
Throw $_
}
Restart-Explorer
} #process
End {
Write-Verbose "[$((Get-Date).TimeofDay) END ] Ending $($myinvocation.mycommand)"
} #end
}
You can see that the functions call the Restart-Explorer function. My version of SearchBarTools.ps1 includes that function. I also decided to have the function write a boolean result to the pipeline. I can use this in control scripts if I want to verify the process.
These commands also support -WhatIf which will be passed on to the Restart-Explorer function.
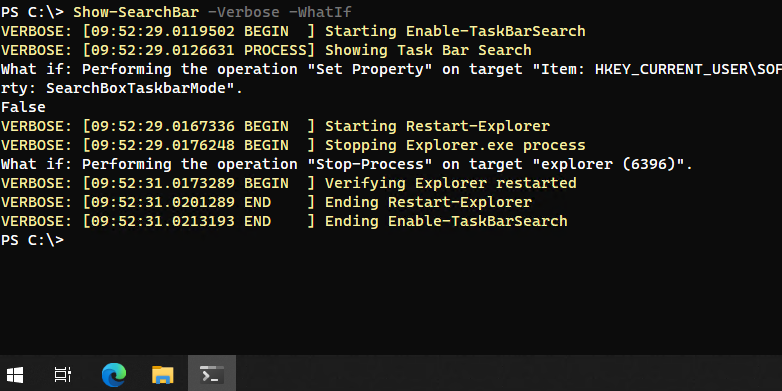
Now I have a set of PowerShell tools that I can use with my desktop automation tools to configure settings exactly as I need them for my coursework.
Hope you found something useful in the code. Enjoy.
Nice one. Now I am ready to dive into the world of [cmdletbinding(SupportsShouldProcess)].