I've been using the PSReadline module for years in PowerShell. I especially loved it when the module added inline command prediction based on your history. You would start typing a command and the module would search your saved history and suggest an inline completion.
ManageEngine ADManager Plus - Download Free Trial
Exclusive offer on ADManager Plus for US and UK regions. Claim now!
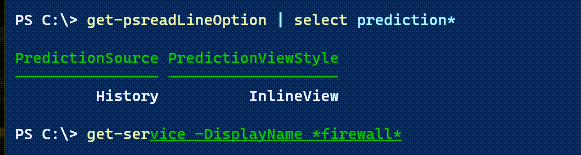
In my PowerShell profile I enable and configure this feature.
Set-PSReadLineOption -PredictionSource History -colors @{
InlinePrediction = "$([char]27)[4;92m"
}
Recently, Microsoft updated the PSReadline module to version 2.2.2. You can install it from the PowerShell Gallery. If you've never updated the module, you will need to actually install it.
Install-Module PSReadline -force
This is because PSReadline ships with PowerShell. Once you've installed a newer version, you can use Update-Module to keep it up to date.
The newer version brings an alternate prediction view style. You can now specify a list view.
Set-PSReadLineOption -PredictionViewStyle ListView
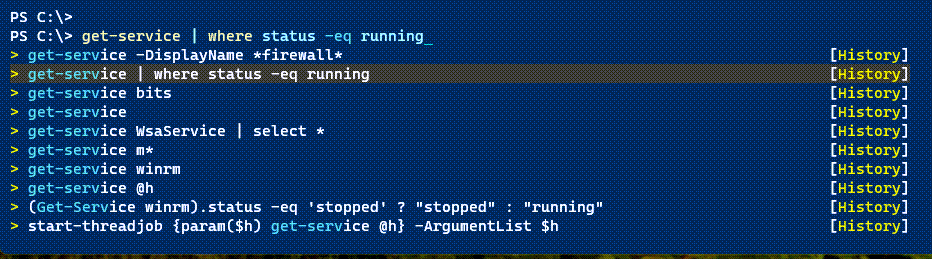
Arrow down to the selection you want, and press Enter. It may not seem like much, but it is little things like this that save time throughout your day and remove a bit of command-line friction.
Toggle Style
What I have found is that sometimes I want inline prediction and sometimes I want a list. But I don't want to be constantly running Set-PSReadlineOption. Although with command prediction, I guess it wouldn't be too bad. Instead, I wrote a PowerShell function that by default toggles the prediction view style.
Function Set-PredictionView {
[cmdletbinding(SupportsShouldProcess)]
[alias("spv", 'sview')]
[OutputType("none")]
Param(
[Parameter(Position = 0, HelpMessage = "Specify the prediction style. Toggle will switch to the unused style.")]
[ValidateSet("List", "InLine", "Toggle")]
[string]$View = "Toggle",
[switch]$Passthru
)
Try {
Switch ($View) {
"List" { $style = "ListView" }
"Inline" { $style = "InLineView" }
"Toggle" {
Switch ((Get-PSReadLineOption).PredictionViewStyle) {
"InLineview" {
$style = "ListView"
}
"ListView" {
$style = "InLineView"
}
Default {
#fail safe action. This should never happen.
Write-Warning "Could not determine a view style. Are you running PSReadline v2.2.2 or later?"
}
} #nested switch
} #toggle
} #switch view
if ($style -AND ($PSCmdlet.ShouldProcess($style, "Set prediction view style"))) {
Set-PSReadLineOption -PredictionViewStyle $style
if ($Passthru) {
Get-PredictionView
}
}
} #try
Catch {
Write-Warning "There was a problem. Could not determine a view style. Are you running PSReadline v2.2.2 or later? $($_.exception.message)."
}
} #end function
This code requires PSReadline version 2.2.2 or later. You can use the function to easily set a view style.
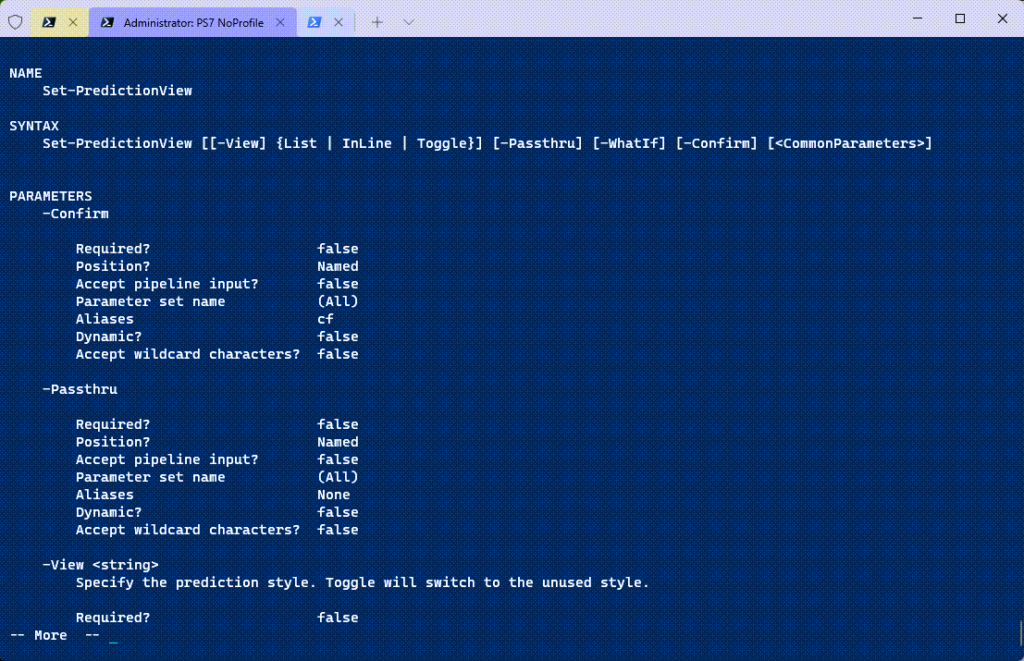
I set the default "style" to toggle between List and Inline. If I'm using List, the function will toggle the style to Inline and vice versa. Or I can explicitly set a style.
Set-predictionview List
Get View Settings
Of course, if I'm setting something I should have a function to get something.
Function Get-PredictionView {
[cmdletbinding()]
[OutputType('PSPredictionView')]
[alias('gview')]
Param()
#define escape character based on PowerShell version
if ($IsCoreCLR) {
$e = '`e'
}
else {
$e = '[char]0x1b)'
}
#get prediction related PSReadline options
$options = Get-PSReadLineOption | Select-Object PredictionSource, PredictionViewStyle, ListPredictionColor,
ListPredictionSelectedColor, InlinePredictionColor
#create a new PSPredictionView object
[PSCustomObject]@{
PSTypeName = "PSPredictionView"
Source = $options.PredictionSource
Style = $options.PredictionViewStyle
ListColor = "{0}$e{1}$([char]27)[0m" -f $options.ListPredictionColor, ($options.ListPredictionColor -replace [char]27, "")
ListSelectedColor = "{0}$e{1}$([char]27)[0m" -f $options.ListPredictionSelectedColor, ($options.ListPredictionSelectedColor -replace [char]27, "")
InlineColor = "{0}$e{1}$([char]27)[0m" -f $options.InlinePredictionColor, ($options.InlinePredictionColor -replace [char]27, "")
}
} #end function
This function is called when running Set-PredictionView -passthru
.
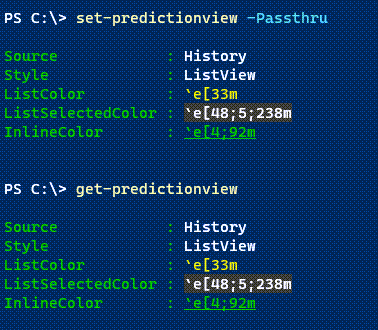
The functions have aliases which make them very easy to use from the console. With only a few keystrokes I can toggle between list and inline views. Talk about reducing friction!
Summary
If you aren't using this PSReadline feature, you are missing out. I am aware that there is a potential security risk because the History option stores commands in a plain text file, so some people choose not to use it. But if you do, I hope you'll give these commands a try and let me know what you think.
Later that day...
I guess Microsoft must have also thought you might want to toggle between views. I never looked, but thanks to friends on Twitter, you can also press F2 to toggle between inline and list prediction views. I guess you don't really need my Set function unless you've re-mapped F2 to something else. You should run Get-PSReadlineKeyHandler to see what else you might be missing out on!
Hi Jeff, thanks for your great article. Not sure you are already aware but by default you can already switch between inline and list view by pressing the F2 key..
This was pointed out to me on Twitter yesterday and I updated the article to make note. I was not aware of the key binding and didn’t even think to check.
Hi there, I am running into an issue which I would like to resolved asap. This is such a useful and a helpful feature but some how in my environment I am unable to run or install the module using Install-Module PSReadline -force
I get an error which states
WARNING: Unable to resolve package source ‘https://www.powershellgallery.com/api
/v2’.
PackageManagement\Install-Package : No match was found for the specified
search criteria and module name ‘PSReadline’. Try Get-PSRepository to see all
available registered module repositories.
At C:\Program
Files\WindowsPowerShell\Modules\PowerShellGet\1.0.0.1\PSModule.psm1:1809
char:21
+ … $null = PackageManagement\Install-Package @PSBoundParameters
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : ObjectNotFound: (Microsoft.Power….InstallPacka
ge:InstallPackage) [Install-Package], Exception
+ FullyQualifiedErrorId : NoMatchFoundForCriteria,Microsoft.PowerShell.Pac
kageManagement.Cmdlets.InstallPackage
First, make sure you are running the latest version of the PowerShellGet module which is version 2.2.5. You might need to upgrade that first. If you are still running v1.0.0.1, which it looks like you are, you’ll need to actually install it. You might also need to set your TLS settings: [Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls12 In fact, I’d run that first then install the latest PwoerShellGet module, then try Install-Module again.
Been waiting for this feature for Windows Powershell for a long time. Thanks a lot!