I have been trying out the latest AI, ChatGPT. I've asked it silly questions.
ManageEngine ADManager Plus - Download Free Trial
Exclusive offer on ADManager Plus for US and UK regions. Claim now!
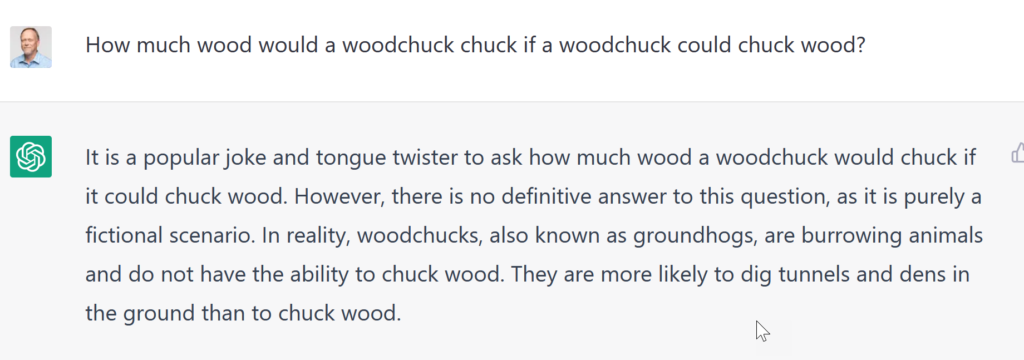
As well as the really important questions.
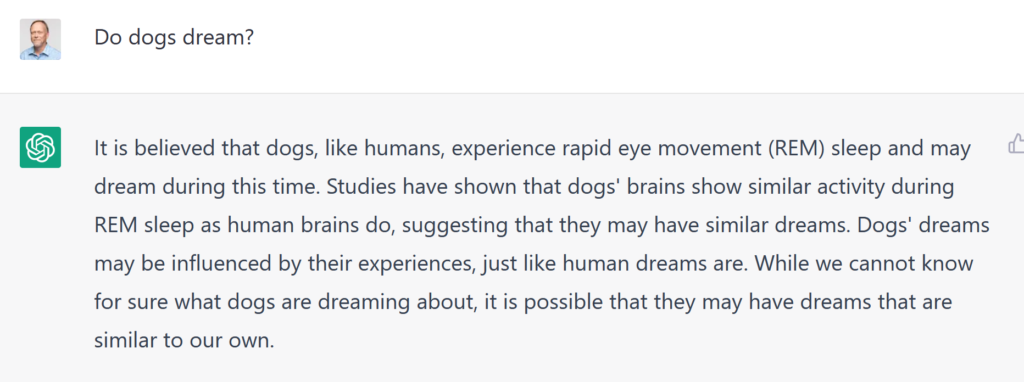
But what I find most fascinating is using ChatGPT to write PowerShell code. I gave the AI this instruction: "Write a PowerShell function that will display "Hello, World" in the center of the screen in alternating colors. Include a parameter to have the message blink.". After a few seconds, it generated this code.
function Show-HelloWorld {
[CmdletBinding()]
param(
[Parameter(Mandatory=$true)]
[ValidateSet("On", "Off")]
[string]$Blink
)
# Clear the screen
Clear-Host
# Set the text colors to alternate between red and green
$colors = @("Red", "Green")
$colorIndex = 0
# Set the cursor position to the center of the screen
$x = (Get-Host).UI.RawUI.WindowSize.Width / 2
$y = (Get-Host).UI.RawUI.WindowSize.Height / 2
# Set the cursor position and display the message
Write-Host "Hello, World" -ForegroundColor $colors[$colorIndex] -NoNewline
$host.UI.RawUI.CursorPosition = New-Object System.Management.Automation.Host.Coordinates $x, $y
# Toggle the text color and blink the message if the Blink parameter is set to "On"
while ($Blink -eq "On") {
Start-Sleep -Milliseconds 500
$colorIndex = ($colorIndex + 1) % 2
Write-Host "`b" -NoNewline
Write-Host "Hello, World" -ForegroundColor $colors[$colorIndex] -NoNewline
}
}
You need to think of this process as scripting with a friend who might still be learning PowerShell. To the AI's credit, it used a proper verb for the function. It was smart enough to realize clearing the screen would be necessary. And the scripting language is what I would expect from someone just getting started. Although, it didn't make my blink option a switch, or even a boolean value. But that is easy enough to change. I'll run it without blinking.
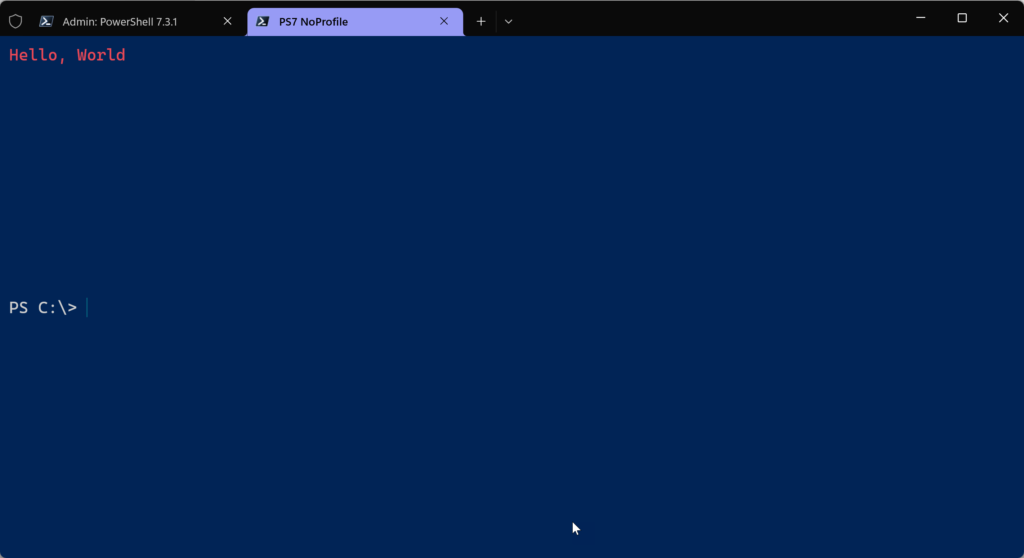
Not quite what I had in mind. Adding blinking also wasn't what I hoped for.
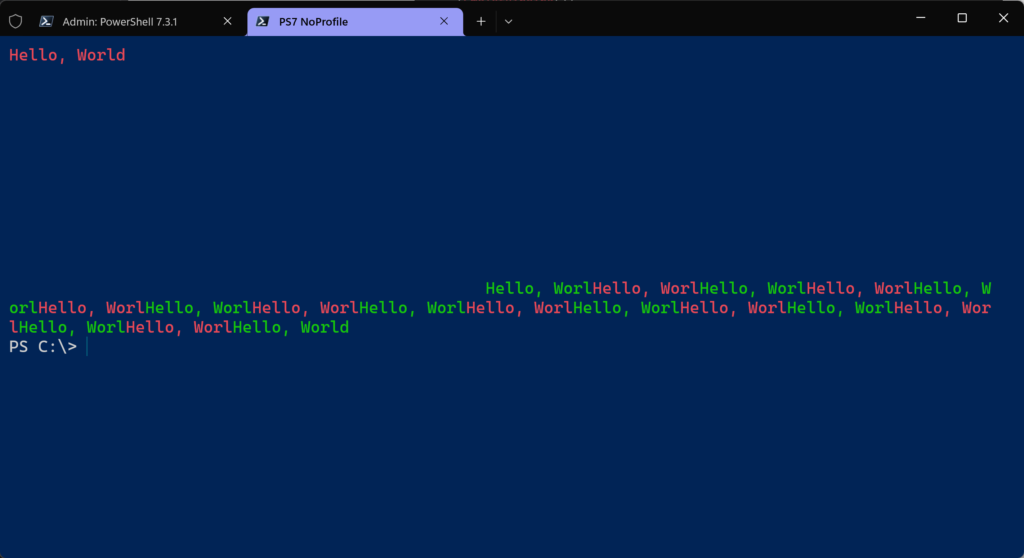
I asked ChatGPT to revise the function.
Revise the function to use ANSI escape sequences or $PSStyle. Each character should be a different color.
The result wasn't much better, and failed to run. Here's an excerpt.
# Set the text colors to alternate between red and green
$colors = @("31", "32")
$colorIndex = 0
# Set the cursor position to the center of the screen
$x = (Get-Host).UI.RawUI.WindowSize.Width / 2
$y = (Get-Host).UI.RawUI.WindowSize.Height / 2
# Set the cursor position and display the message
Write-Host "H" -NoNewline
Write-Host "e" -NoNewline -ForegroundColor $colors[$colorIndex]
$colorIndex = ($colorIndex + 1) % 2
Write-Host "l" -NoNewline -ForegroundColor $colors[$colorIndex]
$colorIndex = ($colorIndex + 1) % 2
Write-Host "l" -NoNewline -ForegroundColor $colors[$colorIndex]
$colorIndex = ($colorIndex + 1) % 2
Write-Host "o" -NoNewline -ForegroundColor $colors[$colorIndex]
$colorIndex = ($colorIndex + 1) % 2
Write-Host ", " -NoNewline -ForegroundColor $colors[$colorIndex]
But I could use the code as a starting point and revise it with what I know about PowerShell. The point I'm making is that using AI, even something like CodePilot, can be a great help, but it won't teach you PowerShell, and you are still responsible for writing effective and proper code.
That said, let me leave you with two more functions I asked ChatGPT to create for me. I wanted a function to display a fractal in the console window. One function creates a Julia set.
function Show-JuliaSet {
[cmdletbinding()]
param (
[double]$cReal = -0.8,
[double]$cImag = 0.156,
[int]$width = 80,
[int]$height = 24,
[int]$maxIterations = 256
)
# Create the Julia set function
function julia {
param (
[double]$cReal,
[double]$cImag,
[int]$width,
[int]$height,
[int]$maxIterations
)
# Calculate the pixel values for the Julia set
$pixels = for ($y = 0; $y -lt $height; $y++) {
for ($x = 0; $x -lt $width; $x++) {
# Map the pixel coordinates to the complex plane
$zReal = [math]::Round((($x / $width) * 3.5) - 2.5, 2)
$zImag = [math]::Round((($y / $height) * 2) - 1, 2)
# Iterate the Julia set equation
$iteration = 0
while (($zReal * $zReal + $zImag * $zImag) -lt 4) {
$tempReal = $zReal * $zReal - $zImag * $zImag + $cReal
$zImag = 2 * $zReal * $zImag + $cImag
$zReal = $tempReal
$iteration++
if ($iteration -ge $maxIterations) {
break
}
}
# Return the number of iterations as a pixel value
$iteration
}
}
# Print the Julia set to the console
for ($y = 0; $y -lt $height; $y++) {
for ($x = 0; $x -lt $width; $x++) {
$pixel = $pixels[$y * $width + $x]
if ($pixel -eq $maxIterations) {
Write-Host "." -NoNewline
} else {
Write-Host " " -NoNewline
}
}
Write-Host ""
}
}
# Call the Julia set function
julia -cReal $cReal -cImag $cImag -width $width -height $height -maxIterations $maxIterations
}
I inverted the original display code.
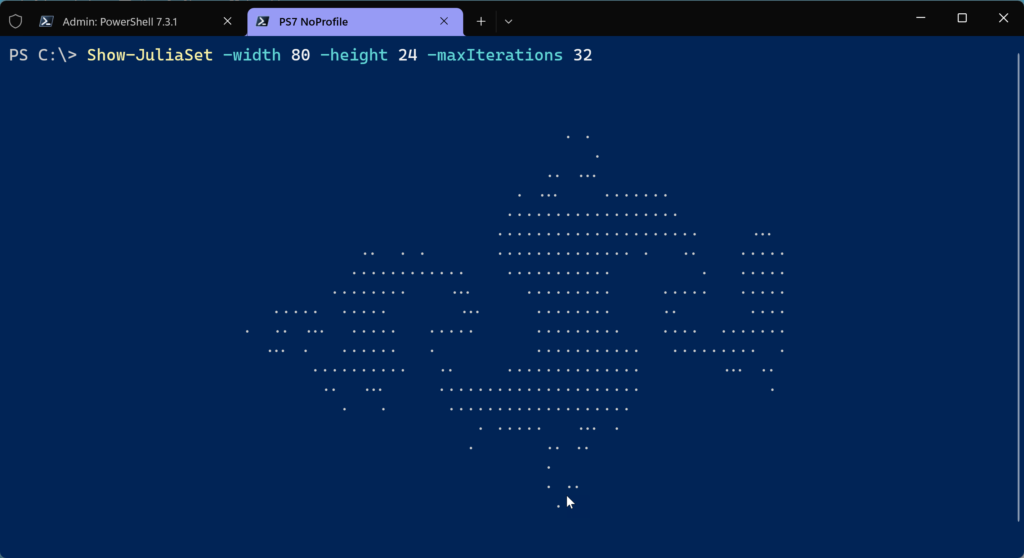
I have to admit, I would never have figured out the math part, and that is definitely true in the Mandelbrot function.
function Show-MandelbrotSet {
[cmdletbinding()]
param (
[int]$width = 80,
[int]$height = 24,
[int]$maxIterations = 256
)
# Create the Mandelbrot set function
function mandelbrot {
param (
[int]$width,
[int]$height,
[int]$maxIterations
)
# Calculate the pixel values for the Mandelbrot set
$pixels = for ($y = 0; $y -lt $height; $y++) {
for ($x = 0; $x -lt $width; $x++) {
# Map the pixel coordinates to the complex plane
$cReal = [math]::Round((($x / $width) * 3.5) - 2.5, 2)
$cImag = [math]::Round((($y / $height) * 2) - 1, 2)
$zReal = 0
$zImag = 0
# Iterate the Mandelbrot set equation
$iteration = 0
while (($zReal * $zReal + $zImag * $zImag) -lt 4) {
$tempReal = $zReal * $zReal - $zImag * $zImag + $cReal
$zImag = 2 * $zReal * $zImag + $cImag
$zReal = $tempReal
$iteration++
if ($iteration -ge $maxIterations) {
break
}
}
# Return the number of iterations as a pixel value
$iteration
}
}
# Print the Mandelbrot set to the console
for ($y = 0; $y -lt $height; $y++) {
for ($x = 0; $x -lt $width; $x++) {
$pixel = $pixels[$y * $width + $x]
if ($pixel -eq $maxIterations) {
Write-Host " " -NoNewline
} else {
Write-Host "." -NoNewline
}
}
Write-Host ""
}
}
# Call the Mandelbrot set function
mandelbrot -width $width -height $height -maxIterations $maxIterations
}
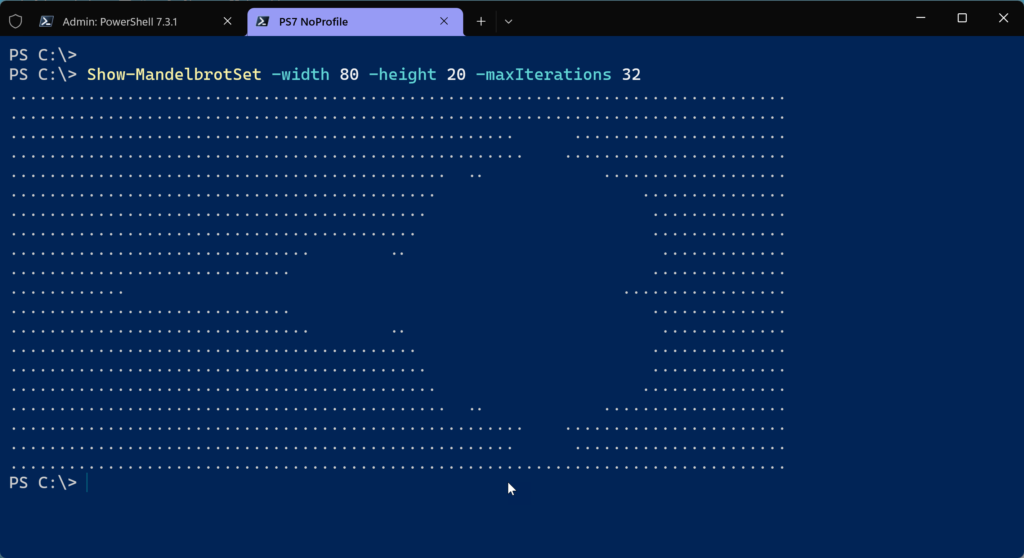
I'll let you take these functions and develop them further.
I find coding with an AI quite fascinating. I definitely don't worry about being replaced any time soon. But the AI can take some of the grunt work from coding and may even offer an approach that you hadn't even considered. Think of the AI as a muse for inspiration.
What have been your experiences scripting an AI or using CodePilot in VSCode?
WOW ,Great article.
Thoughtful article!
Enjoyed how specific shortcomings of the AI were pointed out while acknowledging other strengths in the fractal examples. Well written, as always. Thank you, Jeff.
I use chatgpt as I had to use BASH, it is very helpful to provide working codes. I ask specific tasks, that I then change to meet my requirement. Works most of the time. Saves my time researching.
When asking complex code, I have had less success , so I stick to asking simple tasks. Great post. Echo’s my own experience.